Table Of Content
- Resources
- Respond with standard HTTP Error Codes
- Understanding REST API design- 6 Key constraints every engineer must know
- Our take on microservices
- if(codePromise) return codePromise
- Get started with Postman
- $(".ownership-indicator").addClass('owned');
- Do we really need to move Monolithic to Microservices

Whenever a client sends a request to an API, the API processes the request and returns a response. This process can sometimes take a considerable amount of time if the API has to perform complex database queries or execute resource-intensive operations. Caching helps to speed up this process by storing frequently accessed resources in a cache.
Resources

Once the user's identity has been verified, the API can grant access to the requested resource. In this article, we will explore the benefits of using API design patterns, common patterns used in API development, and best practices for implementing API design patterns. Paths of endpoints should be consistent, we use nouns only since the HTTP methods indicate the action we want to take. Paths of nested resources should come after the path of the parent resource. They should tell us what we’re getting or manipulating without the need to read extra documentation to understand what it’s doing.
Respond with standard HTTP Error Codes
The above REST API design patterns help create simple, scalable, and easy-to-maintain APIs. REST (Representational State Transfer) API design patterns follow best practices and guidelines for web services. If we try to add a new workout but forget to provide the "mode" property in our request body, we should see the error message along with the 400 HTTP error code. When something goes wrong (either from the request or inside our API) we send HTTP Error codes back. I've seen and used API's that were returning all the time a 400 error code when a request was buggy without any specific message about WHY this error occurred or what the mistake was.
Understanding REST API design- 6 Key constraints every engineer must know
To improve the experience we also can send a quick error message along with the error response. But as I've written in the introduction this isn't always very wise and should be considered by the engineer themself. Now you can go to your HTTP client, send the POST request again, and you should receive the newly created workout as JSON. Let's go into our workout service and receive the data inside our createNewWorkout method.
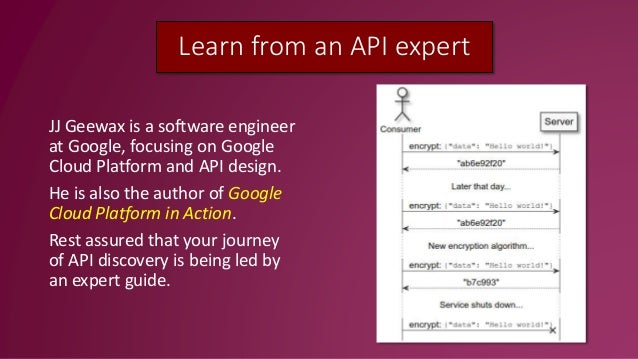
Authentication is the process of verifying the identity of a user or system. In API design, authentication is used to ensure that only authorized users or systems can access the API. This is typically done by requiring users to provide some form of credentials, such as a username and password, an access token, or a digital certificate.
if(codePromise) return codePromise
Serverless Architecture: Five Design Patterns - The New Stack
Serverless Architecture: Five Design Patterns.
Posted: Tue, 07 Mar 2017 08:00:00 GMT [source]
Donations to freeCodeCamp go toward our education initiatives, and help pay for servers, services, and staff. We've defined all the properties that make up a workout including the type and an example. This should look very familiar if you've already worked with API's that have OpenAPI documentation. This is the view where all our endpoints will be listed and you can extend each one to get more information about it. This is basically the whole magic to add an endpoint to our swagger docs.
Get started with Postman
In API design, discoverability and documentation are the unsung heroes. They play a crucial role in simplifying the use of API endpoints and ensuring that developers have the necessary guidance for quick implementation. HTTP specification comes up with a set of standard headers, through which the client can get information about a requested resource and carry the messages that indicate its representations. You may be designing microservices APIs, which have their own set of considerations. Everything covered in this post likely still applies, but you’ll want to pay extra careful attention when designing microservices. Each will need to make sense on its own, yet benefit from a combination (loose coupling).
Although there's the term JavaScript in JSON, it's not tied to it specifically. You can also write your API with Java or Python that can handle JSON as well. There are many different approaches to handling versioning inside an Express API. In our case I'd like to create a sub folder for each version inside our src directory called v1.
When a user requests to view product details, the gateway inspects the request and forwards it to the product catalog service. Similarly, a request to place an order is routed to the order management service. In a microservices architecture, each service has its process and communicates with the other services by a well-defined API which is built on the top of a network. We can add caching to return data from the local memory cache instead of querying the database to get the data every time we want to retrieve some data that users request.
RESTful APIs have become the standard for building web services that are scalable, flexible, and easy to maintain. However, building a successful RESTful API requires careful planning, implementation, and testing. In this answer, we'll cover some of the best practices for building a RESTful API, as well as some common pitfalls to avoid. Where kesh92 is the username of a specific user in the users collection, and will return the location and date of joining for kesh92. These are just some of the ways you could design parameters that strive towards API completion and help your end developers use your API intuitively.
I'm using the shorthand syntax here, to create a new key called "mode" inside the object with the value of whatever is in "req.query.mode". This could be either a truthy value or undefined if there isn't a query parameter called "mode". We can extend this object the more filter parameters we'd like to accept. Now we're able to throw and catch errors in the service and data access layer. We can move into our workout controller now, catch the errors there as well, and respond accordingly.
No comments:
Post a Comment